December 26, 2012
Quick Sort
Divide and Conquer
Comparison of Complexity of Algorithms
N | N2 | N Log2 (N) |
10 | 100 | 33.21 |
100 | 10000 | 664.38 |
1000 | 1000000 | 9965.78 |
10000 | 100000000 | 132877.12 |
100000 | 10000000000 | 1660964.04 |
1000000 | 1E+12 | 19931568.57 |
- Merge Sort
- Quick Sort
- Heap Sort
Bubble Sort
Insertion Sort
Selection Sort
Binary Search
December 25, 2012
Traffic Flow on Nodes in NS2
The output of the above code is as follows. Run the program by typing ‘ns trafficflow.tcl’ in terminal.
After it gets executed, the following output is displayed in nam.
Now, click on the Play button which is underlined green.
We can see the traffic flow as well as the traffic which are being dropped on node 2 because the limits of the node to hold the packets is limited. Thats why when the queue is full and there is no more capacity available, the packet drops.
Code Explained:
set ns [new Simulator]: generates an NS simulator object instance, and assigns it to variable ns.
The "Simulator" object has member functions that do the following:
- Create compound objects such as nodes and links (described later)
- Connect network component objects created (ex. attach-agent)
- Set network component parameters (mostly for compound objects)
- Create connections between agents (ex. make connection between a "tcp" and "sink")
- Specify NAM display options
Most of member functions are for simulation setup (referred to as plumbing functions in the Overview section) and scheduling, however some of them are for the NAM display. The "Simulator" object member function implementations are located in the "ns-2/tcl/lib/ns-lib.tcl" file.
- $ns color fid color: is to set color of the packets for a flow specified by the flow id (fid). This member function of "Simulator" object is for the NAM display, and has no effect on the actual simulation.
- $ns namtrace-all file-descriptor: This member function tells the simulator to record simulation traces in NAM input format. It also gives the file name that the trace will be written to later by the command $ns flush-trace. Similarly, the member function trace-all is for recording the simulation trace in a general format.
- proc finish {}: is called after this simulation is over by the command $ns at 5.0 "finish". In this function, post-simulation processes are specified.
- set n0 [$ns node]: The member function node creates a node. A node in NS is compound object made of address and port classifiers (described in a later section). Users can create a node by separately creating an address and a port classifier objects and connecting them together. However, this member function of Simulator object makes the job easier. To see how a node is created, look at the files: "ns-2/tcl/libs/ns-lib.tcl" and "ns-2/tcl/libs/ns-node.tcl".
- $ns duplex-link node1 node2 bandwidth delay queue-type: creates two simplex links of specified bandwidth and delay, and connects the two specified nodes. In NS, the output queue of a node is implemented as a part of a link, therefore users should specify the queue-type when creating links. In the above simulation script, DropTail queue is used. If the reader wants to use a RED queue, simply replace the word DropTail with RED. The NS implementation of a link is shown in a later section. Like a node, a link is a compound object, and users can create its sub-objects and connect them and the nodes. Link source codes can be found in "ns-2/tcl/libs/ns-lib.tcl" and "ns-2/tcl/libs/ns-link.tcl" files. One thing to note is that you can insert error modules in a link component to simulate a lossy link (actually users can make and insert any network objects). Refer to the NS documentation to find out how to do this.
- $ns queue-limit node1 node2 number: This line sets the queue limit of the two simplex links that connect node1 and node2 to the number specified. At this point, the authors do not know how many of these kinds of member functions of Simulator objects are available and what they are. Please take a look at "ns-2/tcl/libs/ns-lib.tcl" and "ns-2/tcl/libs/ns-link.tcl", or NS documentation for more information.
- $ns duplex-link-op node1 node2 ..: The next couple of lines are used for the NAM display. To see the effects of these lines, users can comment these lines out and try the simulation.
Now that the basic network setup is done, the next thing to do is to setup traffic agents such as TCP and UDP, traffic sources such as FTP and CBR, and attach them to nodes and agents respectively.
- set tcp [new Agent/TCP]: This line shows how to create a TCP agent. But in general, users can create any agent or traffic sources in this way. Agents and traffic sources are in fact basic objects (not compound objects), mostly implemented in C++ and linked to OTcl. Therefore, there are no specific Simulator object member functions that create these object instances. To create agents or traffic sources, a user should know the class names these objects (Agent/TCP, Agnet/TCPSink, Application/FTP and so on). This information can be found in the NS documentation or partly in this documentation. But one shortcut is to look at the "ns-2/tcl/libs/ns-default.tcl" file. This file contains the default configurable parameter value settings for available network objects. Therefore, it works as a good indicator of what kind of network objects are available in NS and what are the configurable parameters.
- $ns attach-agent node agent: The attach-agent member function attaches an agent object created to a node object. Actually, what this function does is call the attach member function of specified node, which attaches the given agent to itself. Therefore, a user can do the same thing by, for example, $n0 attach $tcp. Similarly, each agent object has a member function attach-agent that attaches a traffic source object to itself.
- $ns connect agent1 agent2: After two agents that will communicate with each other are created, the next thing is to establish a logical network connection between them. This line establishes a network connection by setting the destination address to each others' network and port address pair.
Assuming that all the network configuration is done, the next thing to do is write a simulation scenario (i.e. simulation scheduling). The Simulator object has many scheduling member functions. However, the one that is mostly used is the following:
- $ns at time "string": This member function of a Simulator object makes the scheduler (scheduler_ is the variable that points the scheduler object created by [new Scheduler] command at the beginning of the script) to schedule the execution of the specified string at given simulation time. For example, $ns at 0.1 "$cbr start" will make the scheduler call a start member function of the CBR traffic source object, which starts the CBR to transmit data. In NS, usually a traffic source does not transmit actual data, but it notifies the underlying agent that it has some amount of data to transmit, and the agent, just knowing how much of the data to transfer, creates packets and sends them.
After all network configuration, scheduling and post-simulation procedure specifications are done, the only thing left is to run the simulation. This is done by $ns run.
Nodes creation in NS2
Add the following code to the new file and named it with the extension of tcl. i.e. filename.tcl . To create the file go to the terminal.
Write
gedit filename.tcl
This above command will open a file with the name filename.tcl. Add the following code to the file and save the file.
Now, in order to run the file go to the Terminal and write the following command.
ns filename.tcl
Code:
#Create a simulator object
set ns [new Simulator]
#Open the NAM trace file
set nf [open o.nam w]
$ns namtrace-all $nf
# create nodes
set n0 [$ns node]
set n1 [$ns node]
set n2 [$ns node]
set n3 [$ns node]
set n4 [$ns node]
set n5 [$ns node]
set n7 [$ns node]
set n8 [$ns node]
set n10 [$ns node]
December 19, 2012
NS2 and UBUNTU Installation on VMWARE
December 10, 2012
Voice over IP (VOIP) on packet tracer
Router(dhcp-config)#option 150 ip 192.168.1.1
Router(dhcp-config)#exit
Router(config)#telephony-service
Router(config-telephony)#max-dn 5
Router(config-telephony)#max-ephones 5
Router(config-telephony)#ip source-address 192.168.1.1 port 2000
Router(config-telephony)#auto assign 1 to 9
Router(config-telephony)#exit
Router(config)#ephone-dn 1
%LINK-3-UPDOWN: Interface ephone_dsp DN 1.1, changed state to up
Router(config-ephone-dn)#number 12345
Router(config-ephone-dn)#exit
%IPPHONE-6-REGISTER: ephone-1 IP:192.168.1.2 Socket:2 DeviceType:Phone has registered.
Router(config-ephone-dn)#number 123
Router(config-ephone-dn)#exit
%LINK-3-UPDOWN: Interface ephone_dsp DN 3.1, changed state to up
Router(config-ephone-dn)#number 11111
Router(config-ephone-dn)#exit
Switch(config-if-range)#switchport voice vlan 1
December 9, 2012
Telnet and SSH on packet tracer
a network protocol that ensures a high-level encryption, allowing for the data transmitted over insecure networks, such as the Internet, to be kept intact and integrate. SSH and SSH Telnet, in particular, work for establishing a secure communication between two network-connected computers as an alternative to remote shells, such as TELNET, that send sensitive information in an insecure environment.
In contrast to other remote access protocols, such as FTP, SSH Telnet ensures higher level of connection security between distant machines but at the same time represents a potential threat to the server stability. Thus, SSH access is considered a special privilege by hosting providers and is often assigned to users only per request.
WORD Default domain nameSwitch(config)#ip domain name abc.comSwitch(config)#crypto key generate rsa
% Please define a hostname other than Switch.
Switch(config)#hostname s1
s1(config)#ip domain name cs-studys1
s1(config)#crypto key generate rsa
Choose the size of the key modulus in the range of 360 to 2048 for your General Purpose Keys. Choosing a key modulus greater than 512 may take a few minutes.
s1(config)#line vty 0 15
s1(config-line)#transport input ssh
The name for the keys will be: s1.cs-study
% Generating 1024 bit RSA keys, keys will be non-exportable...[OK]
s1(config)#ip ssh version 2
s1(config-line)#
Password Authentication Protocol on packet tracer (PAP)
A password authentication protocol (PAP) is an authentication protocol that uses a password. PAP is used by Point to Point Protocol to validate users before allowing them access to server resources. Almost all network operating system remote servers support PAP.
PAP transmits unencrypted ASCII passwords over the network and is therefore considered insecure. It is used as a last resort when the remote server does not support a stronger authentication protocol, like CHAP or EAP (the latter is actually a framework).
Password-based authentication is the protocol that two entities share a password in advance and use the password as the basis of authentication. Existing password authentication schemes can be categorized into two types: weak-password authentication schemes and strong-password authentication schemes. In general, strong-password authentication protocols have the advantages over the weak-password authentication schemes in that their computational overhead are lighter, designs are simpler, and implementation are easier, and therefore are especially suitable for some constrained environments.
PAP works basically the same way as the normal login procedure. The client authenticates itself by sending a user name and an (optionally encrypted) password to the server, which the server compares to its secrets database. This technique is vulnerable to eavesdroppers who may try to obtain the password by listening in on the serial line, and to repeated trial and error attacks.
Let us apply PPP on packet tracer. Consider the following simpler topology.
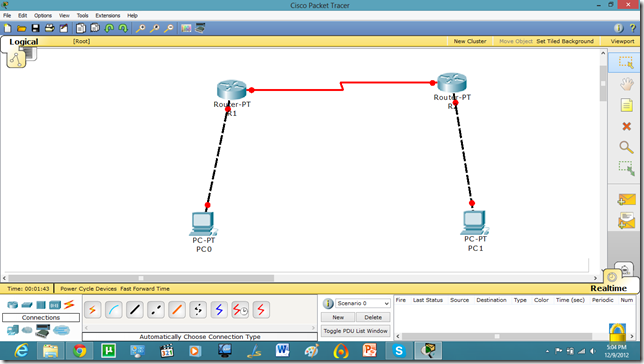
Let us apply IP addresses on the interfaces and change the state of the interface from down to UP. So that they can communicate.
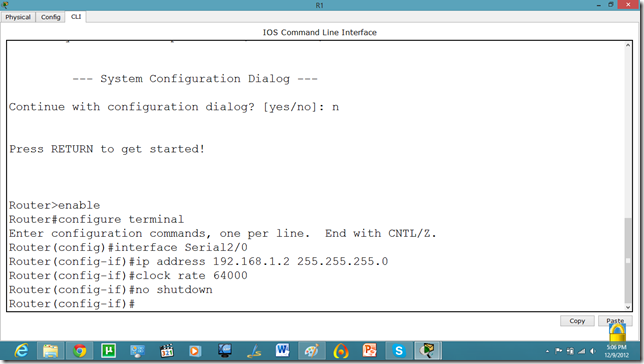
Similarly, for serial interface.
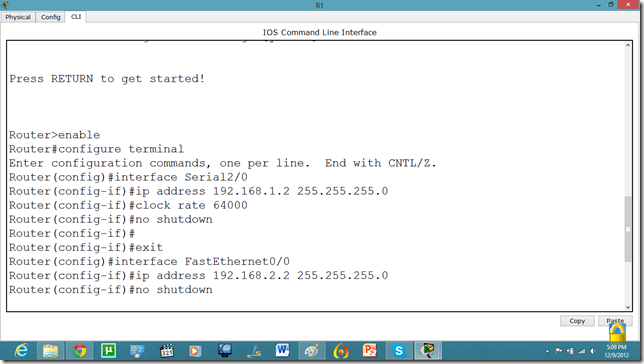
PC IP setup
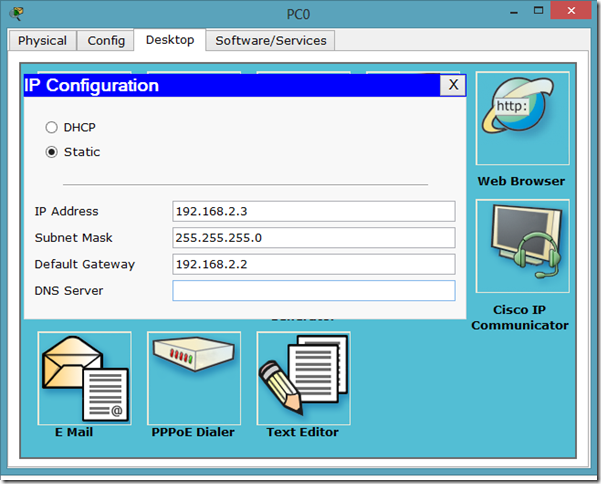
The IP configuration on other router.

serial int setup.
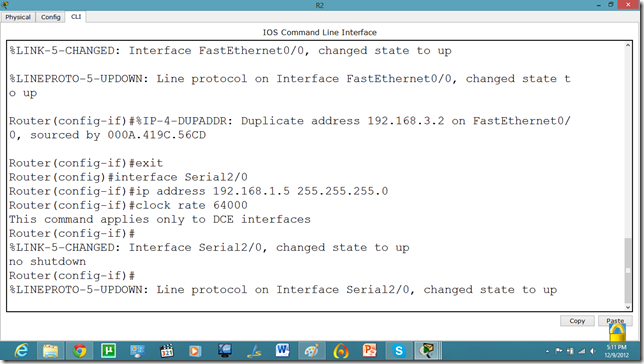
Now, we know that PCs that are attached cannot communicate until we apply a routing mechanism. In this case we are applying the RIP V2 protocol. Apply the following set of commands on both routers. We have also set the hostname of the router which will be useful to us later.
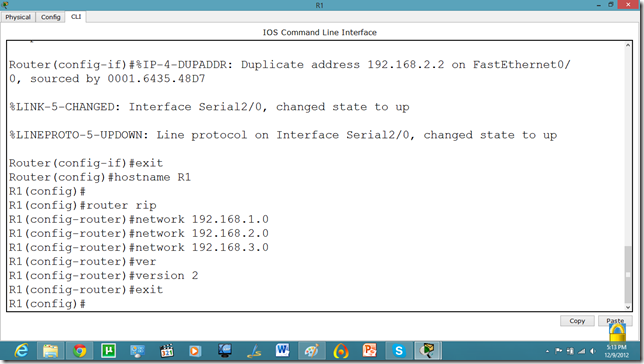
Now, let us set the commands on the second router as well.
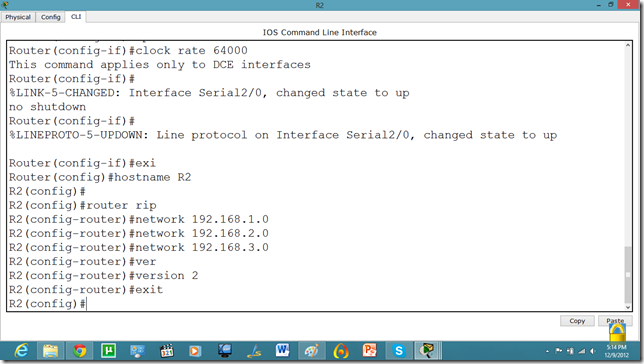
Now, both PCs can communicate.
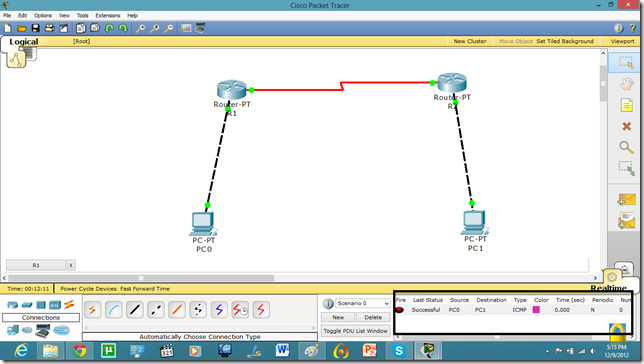
Now, we will set the authentication, In this tutorial we are going to apply PAP.
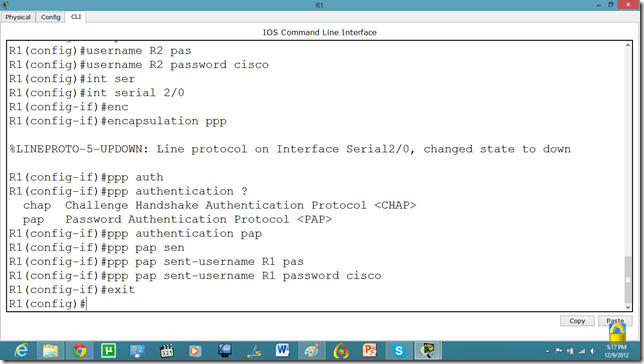
As we set the authentication on one router the communication is disabled.
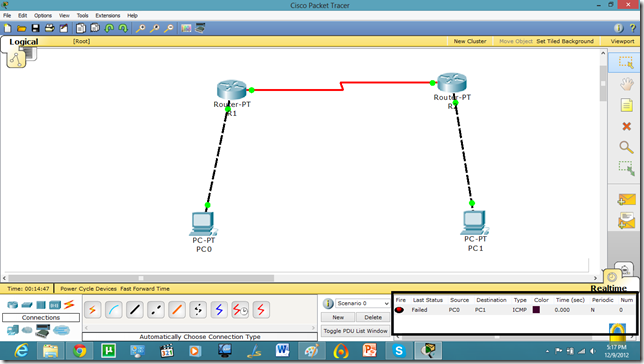
Let us set it on other router as well.
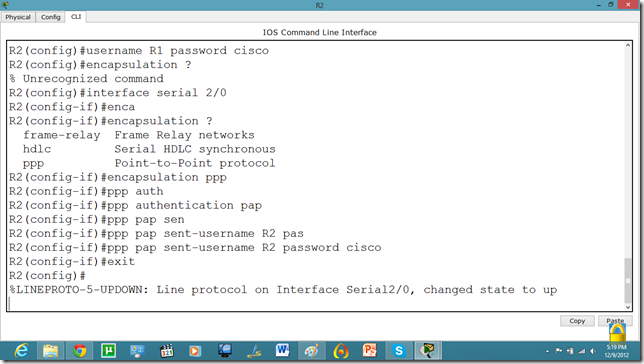
Now, they can communicate.
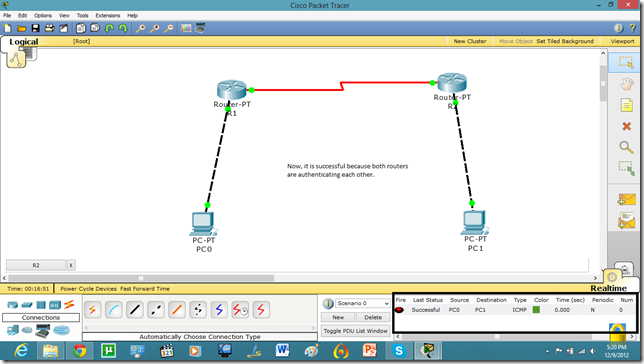
Now, if we run show run command in enable mode. We can see the authentication enabled in router.
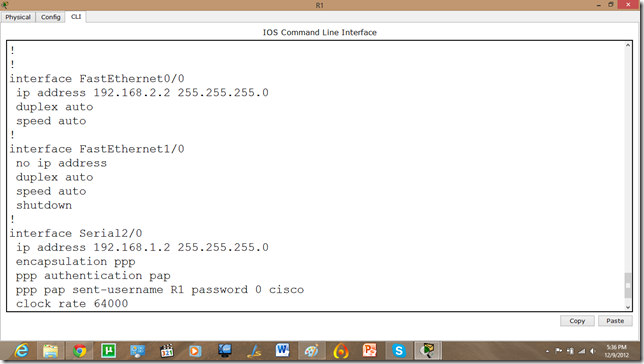
C program to Read From a File
#include <stdio.h> #include <stdlib.h> void main() { FILE *fptr; char filename[15]; char ch; ...
-
A terminal emulation program for TCP/IP networks such as the Internet. The Telnet program runs on your computer and connects your PC to a ...
-
Voice over IP (VoIP, or voice over Internet Protocol) commonly refers to the communication protocols, technologies, methodologies, and tra...
-
Star Formations http://cs-study.blogspot.com/2013/10/c-program-to-print-different-star.html Hollow...